We have a custom assembly for data types and custom steps that go into the FlowWright directory. In both cases, they call back to the application’s REST API. You may foresee some difficulties with a few dependencies.
Most Microsoft examples use dependency injection to inject an instance of IHttpClientFactory.
Here's an example of using the HTTP Client to access data types and/or process steps with an HTTP context.
using System;
using System.Net.Http;
using System.Threading.Tasks;
namespace HttpClientExample
{
class Program
{
static async Task Main(string[] args)
{
using (HttpClient client = new HttpClient())
{
try
{
HttpResponseMessage response = await client.GetAsync("https://api.example.com/data");
response.EnsureSuccessStatusCode();
string responseBody = await response.Content.ReadAsStringAsync();
Console.WriteLine(responseBody);
}
catch (HttpRequestException e)
{
Console.WriteLine($"Request error: {e.Message}");
}
}
}
}
}
Here's a recommended method to create an instance of HttpClient in FlowWright v10.x.
Create a folder under the c:\FlowWright directory and place your dll there. Then, configure that directory within the appsettings.json file; there is an item called “CustomUIDllsFolder,” put the name of your folder there, and dlls will be automatically loaded when the app starts.
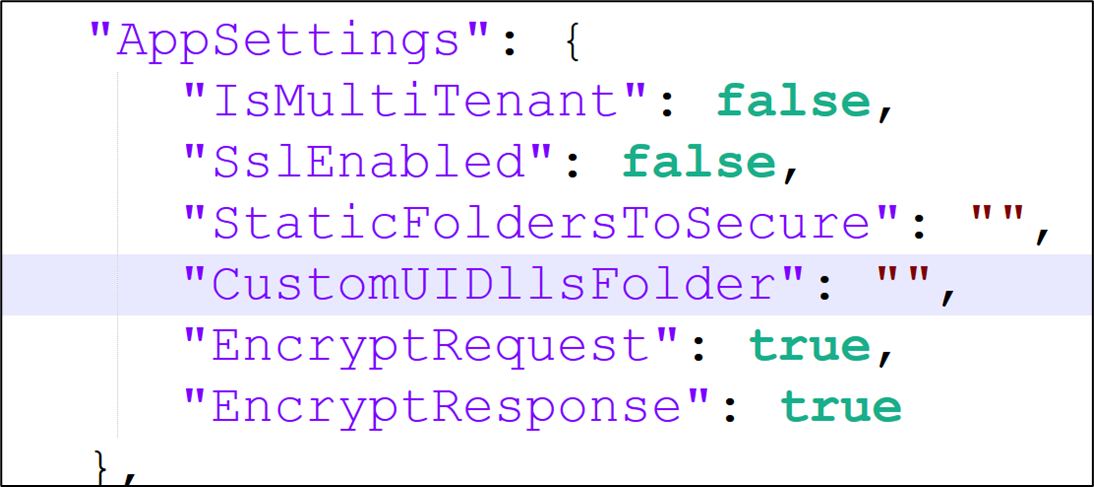